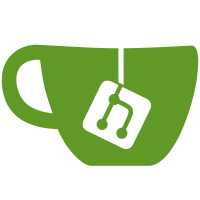
* Work on raft backend * Add logstore locally * Add encryptor and unsealable interfaces * Add clustering support to raft * Remove client and handler * Bootstrap raft on init * Cleanup raft logic a bit * More raft work * Work on TLS config * More work on bootstrapping * Fix build * More work on bootstrapping * More bootstrapping work * fix build * Remove consul dep * Fix build * merged oss/master into raft-storage * Work on bootstrapping * Get bootstrapping to work * Clean up FMS and node-id * Update local node ID logic * Cleanup node-id change * Work on snapshotting * Raft: Add remove peer API (#906) * Add remove peer API * Add some comments * Fix existing snapshotting (#909) * Raft get peers API (#912) * Read raft configuration * address review feedback * Use the Leadership Transfer API to step-down the active node (#918) * Raft join and unseal using Shamir keys (#917) * Raft join using shamir * Store AEAD instead of master key * Split the raft join process to answer the challenge after a successful unseal * get the follower to standby state * Make unseal work * minor changes * Some input checks * reuse the shamir seal access instead of new default seal access * refactor joinRaftSendAnswer function * Synchronously send answer in auto-unseal case * Address review feedback * Raft snapshots (#910) * Fix existing snapshotting * implement the noop snapshotting * Add comments and switch log libraries * add some snapshot tests * add snapshot test file * add TODO * More work on raft snapshotting * progress on the ConfigStore strategy * Don't use two buckets * Update the snapshot store logic to hide the file logic * Add more backend tests * Cleanup code a bit * [WIP] Raft recovery (#938) * Add recovery functionality * remove fmt.Printfs * Fix a few fsm bugs * Add max size value for raft backend (#942) * Add max size value for raft backend * Include physical.ErrValueTooLarge in the message * Raft snapshot Take/Restore API (#926) * Inital work on raft snapshot APIs * Always redirect snapshot install/download requests * More work on the snapshot APIs * Cleanup code a bit * On restore handle special cases * Use the seal to encrypt the sha sum file * Add sealer mechanism and fix some bugs * Call restore while state lock is held * Send restore cb trigger through raft log * Make error messages nicer * Add test helpers * Add snapshot test * Add shamir unseal test * Add more raft snapshot API tests * Fix locking * Change working to initalize * Add underlying raw object to test cluster core * Move leaderUUID to core * Add raft TLS rotation logic (#950) * Add TLS rotation logic * Cleanup logic a bit * Add/Remove from follower state on add/remove peer * add comments * Update more comments * Update request_forwarding_service.proto * Make sure we populate all nodes in the followerstate obj * Update times * Apply review feedback * Add more raft config setting (#947) * Add performance config setting * Add more config options and fix tests * Test Raft Recovery (#944) * Test raft recovery * Leave out a node during recovery * remove unused struct * Update physical/raft/snapshot_test.go * Update physical/raft/snapshot_test.go * fix vendoring * Switch to new raft interface * Remove unused files * Switch a gogo -> proto instance * Remove unneeded vault dep in go.sum * Update helper/testhelpers/testhelpers.go Co-Authored-By: Calvin Leung Huang <cleung2010@gmail.com> * Update vault/cluster/cluster.go * track active key within the keyring itself (#6915) * track active key within the keyring itself * lookup and store using the active key ID * update docstring * minor refactor * Small text fixes (#6912) * Update physical/raft/raft.go Co-Authored-By: Calvin Leung Huang <cleung2010@gmail.com> * review feedback * Move raft logical system into separate file * Update help text a bit * Enforce cluster addr is set and use it for raft bootstrapping * Fix tests * fix http test panic * Pull in latest raft-snapshot library * Add comment
93 lines
3.0 KiB
Go
93 lines
3.0 KiB
Go
package command
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
|
|
log "github.com/hashicorp/go-hclog"
|
|
"github.com/hashicorp/vault/vault"
|
|
vaultseal "github.com/hashicorp/vault/vault/seal"
|
|
shamirseal "github.com/hashicorp/vault/vault/seal/shamir"
|
|
"github.com/pkg/errors"
|
|
)
|
|
|
|
var (
|
|
onEnterprise = false
|
|
)
|
|
|
|
func adjustCoreForSealMigration(logger log.Logger, core *vault.Core, barrierSeal, unwrapSeal vault.Seal) error {
|
|
existBarrierSealConfig, existRecoverySealConfig, err := core.PhysicalSealConfigs(context.Background())
|
|
if err != nil {
|
|
return fmt.Errorf("Error checking for existing seal: %s", err)
|
|
}
|
|
|
|
// If we don't have an existing config or if it's the deprecated auto seal
|
|
// which needs an upgrade, skip out
|
|
if existBarrierSealConfig == nil || existBarrierSealConfig.Type == vaultseal.HSMAutoDeprecated {
|
|
return nil
|
|
}
|
|
|
|
if unwrapSeal == nil {
|
|
// We have the same barrier type and the unwrap seal is nil so we're not
|
|
// migrating from same to same, IOW we assume it's not a migration
|
|
if existBarrierSealConfig.Type == barrierSeal.BarrierType() {
|
|
return nil
|
|
}
|
|
|
|
// If we're not coming from Shamir, and the existing type doesn't match
|
|
// the barrier type, we need both the migration seal and the new seal
|
|
if existBarrierSealConfig.Type != vaultseal.Shamir && barrierSeal.BarrierType() != vaultseal.Shamir {
|
|
return errors.New(`Trying to migrate from auto-seal to auto-seal but no "disabled" seal stanza found`)
|
|
}
|
|
} else {
|
|
if unwrapSeal.BarrierType() == vaultseal.Shamir {
|
|
return errors.New("Shamir seals cannot be set disabled (they should simply not be set)")
|
|
}
|
|
}
|
|
|
|
var existSeal vault.Seal
|
|
var newSeal vault.Seal
|
|
|
|
if existBarrierSealConfig.Type == barrierSeal.BarrierType() {
|
|
// In this case our migration seal is set so we are using it
|
|
// (potentially) for unwrapping. Set it on core for that purpose then
|
|
// exit.
|
|
core.SetSealsForMigration(nil, nil, unwrapSeal)
|
|
return nil
|
|
}
|
|
|
|
if existBarrierSealConfig.Type != vaultseal.Shamir && existRecoverySealConfig == nil {
|
|
return errors.New(`Recovery seal configuration not found for existing seal`)
|
|
}
|
|
|
|
switch existBarrierSealConfig.Type {
|
|
case vaultseal.Shamir:
|
|
// The value reflected in config is what we're going to
|
|
existSeal = vault.NewDefaultSeal(shamirseal.NewSeal(logger.Named("shamir")))
|
|
newSeal = barrierSeal
|
|
newBarrierSealConfig := &vault.SealConfig{
|
|
Type: newSeal.BarrierType(),
|
|
SecretShares: 1,
|
|
SecretThreshold: 1,
|
|
StoredShares: 1,
|
|
}
|
|
newSeal.SetCachedBarrierConfig(newBarrierSealConfig)
|
|
newSeal.SetCachedRecoveryConfig(existBarrierSealConfig)
|
|
|
|
default:
|
|
if onEnterprise && barrierSeal.BarrierType() == vaultseal.Shamir {
|
|
return errors.New("Migrating from autoseal to Shamir seal is not currently supported on Vault Enterprise")
|
|
}
|
|
|
|
// If we're not cominng from Shamir we expect the previous seal to be
|
|
// in the config and disabled.
|
|
existSeal = unwrapSeal
|
|
newSeal = barrierSeal
|
|
newSeal.SetCachedBarrierConfig(existRecoverySealConfig)
|
|
}
|
|
|
|
core.SetSealsForMigration(existSeal, newSeal, unwrapSeal)
|
|
|
|
return nil
|
|
}
|